Build Smart Contracts in Vyper with Truffle 5
The release of Truffle v5 included Vyper support, and today we will explore how we can build a Smart Contract and deploy it on Ethereum’s MainNet.
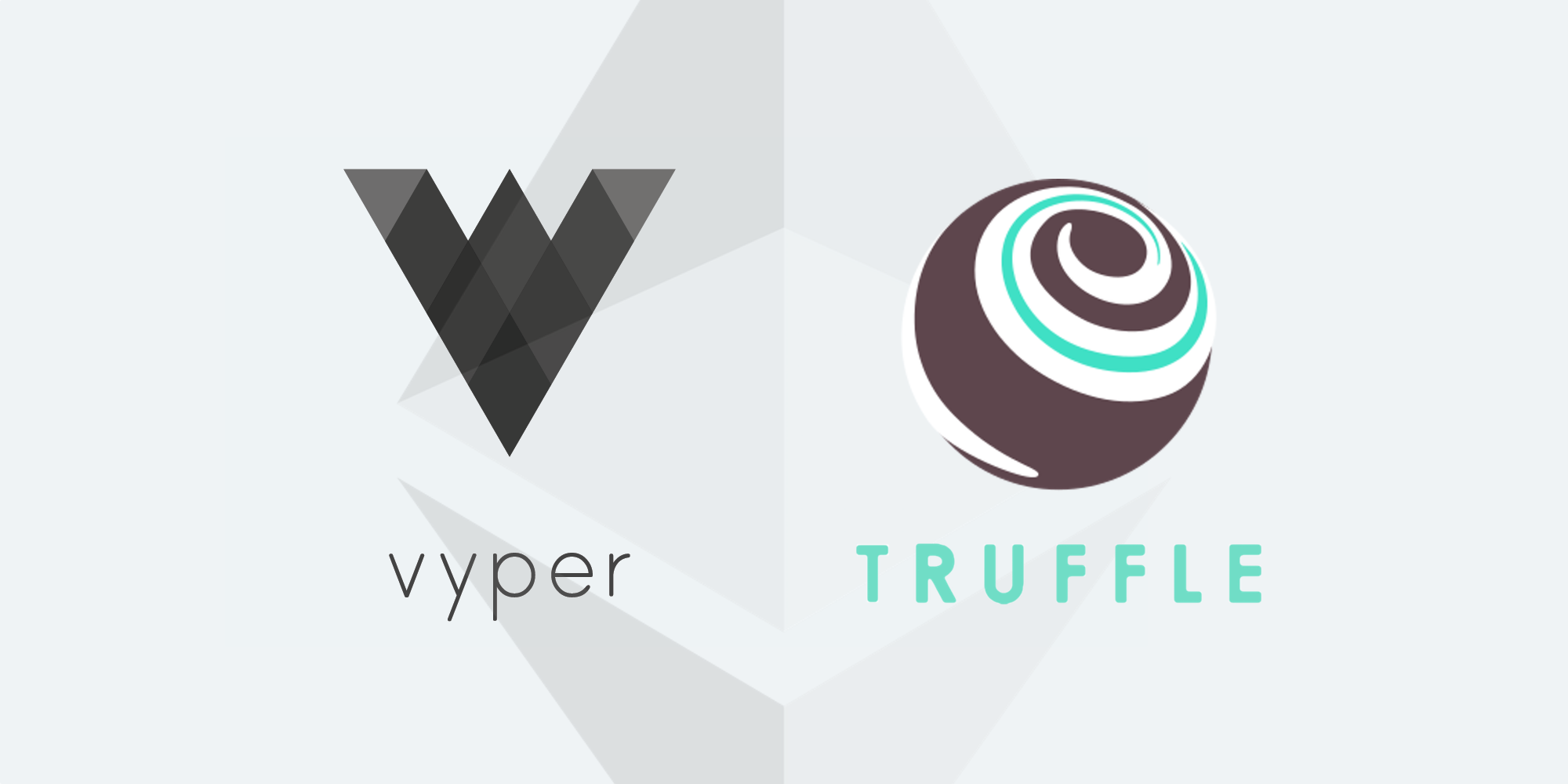
An overview & demo of the Vyper language
The release of Truffle v5 included Vyper support, and today we will explore how we can build a Smart Contract and deploy it on Ethereum’s MainNet.
Building an efficient smart contract language is a billion-dollar problem. Multiple smart contract languages are at the bleeding edge of innovation in competition to become mainstream. In addition to the well-known “Solidity”, there are several experimental languages targeting Ethereum:
- Bamboo — A morphing smart contract language (Not actively developed)
- Vyper — New experimental pythonic programming language (Actively developed)
- LLL — Low-level Lisp-like Language (Not actively developed)
- Flint — New language under development with security features including asset types, state transition, and safe integers (Actively developed)
- Pyramid — A dialect of the Scheme programming language that targets the Ethereum Virtual Machine (EVM). (Not actively developed)
What is Vyper?
Vyper is an experimental smart contract language. It aims for simplicity, human readability, and security. Also, Vyper’s syntax resembles Python.
“Vyper is a contract-oriented, pythonic programming language that targets the Ethereum Virtual Machine (EVM)”
Language Goals of Vyper
Simplicity — Vyper focuses on simplicity and maximizes the chances of writing error-free code. Its compiler implementation also strives for simplicity.
Security & Audibility — Building secure contract comes naturally, that's what Vyper aims for; the code should have a natural reading flow for any reader. That's why Vyper encourages building one-page smart contracts. Code readability provides for better code audibility.
Vyper Vs. Solidity
Vyper’s goals as a language are different than those of Solidity. To achieve simplicity, readability, and audibility, Vyper doesn’t include some of Solidity’s features such as Modifiers and Inheritance. In addition, Vyper also doesn’t include:
- Function overloading
- Operator overloading (Solidity also doesn't support it as of 17 Jan 2018)
- Recursive calling
- Infinite-length loops
- Inline assembly
- Binary fixed point
Vyper scarifies all of the above to achieve it's design goals. However, Vyper does provide some interesting features :
- Bounds and overflow checking: On array accesses as well as on arithmetic level, which improves performance and increases reliability and security.
- Support for signed integers and decimal fixed point numbers
- Decidability: It should be possible to compute a precise upper bound for the gas consumption of any function call.
- Strong typing: Including support for units (e.g. timestamp, timedelta, seconds, wei, wei per second, meters per second squared).
- Small and understandable compiler code
- Limited support for pure functions: Anything marked constant is not allowed to change the state.
Among the above, decidability is a very important feature which you can use to estimate your upper bound of gas usage. This can help in gas optimization, which is a very important part of a smart contract platform.
Installing Vyper
Let’s get to it and install Vyper now. If you are running MacOS, check instructions here (I am running Ubuntu 16.10).
Note- Vyper is in active development, so don’t lose patience if you get installation errors. 😃
Install Python
sudo apt-get update
sudo apt-get install python3.6
sudo apt-get install python3-dev
Create Virtual Environment
If you have worked with Python before then you must know about Virtual Python environment. It is strongly recommended to install Vyper in a Virtual Python environment.
To create a new virtual environment for Vyper run the following commands:
sudo apt install virtualenv
virtualenv -p python3.6 --no-site-packages ~/vyper-venv
source ~/vyper-venv/bin/activate
You must still be in the Virtual environment… now let’s install Vyper:
git clone https://github.com/ethereum/vyper.git
cd vyper
make
make test
If everything went fine, then first thank to Byte God 😃 and compile an example contract:
vyper examples/crowdfund.vy
There are many examples in the example folder of the installation directory. We’ll be going deeper into Vyper usage in future articles, so remember to hit subscribe below!
Truffle with Vyper
Truffle 5 launched recently and it includes Vyper support. So let's upgrade to latest version and provide a Vyper example Box (Truffle boxes, remember?):
npm uninstall -g truffle
npm install truffle
Now let’s download our vyper-exmaple
Truffle Box. Run the command below in an empty repository:
truffle unbox vyper-example
Let’s run our first Vyper Contract. Get into the vyper-example
directory and open truffle console:
cd vyper-example
truffle develop
Now let’s compile our contract and deploy:
compile
migrate
Now let’s see our first Vyper smart contact:
stored_data: uint256
@public
def set(new_value : uint256):
self.stored_data = new_value
@public
@constant
def get() -> uint256:
return self.stored_data
It’s a very basic Vyper smart contract, which is simply storing a state variable in stored_data
and two public get
and set
method. @public
tells that the method is public, and @constant
is a sort of pure
modifier in Solidity which tells that method doesn't change any state. Let's test our smart contact on the same Truffle console:
VyperStorage.deployed().then((token) => {contract = token;}
contract.set(1)
contract.get(0)
Deploy To MainNet using QuickNode
Now let’s deploy our smart contract on MainNet using our own QuickNode. First, we have to install HDWalletProvider to handle the transaction signing as well as the connection to the Ethereum network.
$ npm install truffle-hdwallet-provider
Next, in truffle.js we have to setup our wallet provider (using QuickNode Web3 URL):
var HDWalletProvider = require("truffle-hdwallet-provider");
var mnemonic = "YOUR_MEMONICS"; // use a funded wallet
module.exports = {
networks: {
development: {
host: "127.0.0.1",
port: 7545,
network_id: "*" // Match any network id }, kovan: { provider: function() { return new HDWalletProvider(mnemonic,
"https://mistakenly-smart-jay.quiknode.io/86d9e35e-8cdb-47ad-80a4-84f9e9537afa/C0_tKUunhUc0rM_i1HMxHA==/")
},
network_id: 42
}
}
};
That’s it, you ran and deployed your first Vyper smart contract using truffle!
In future blogs, we will go more in-depth about the Vyper language and building more complex smart contracts.
Awesome Vyper 🐍
Vyper is an experimental language but the community is quite active and building interesting tools (check the full list here). Vyper takes inspiration from Python’s simplicity goals, and can help new Web3 developers understand and build better smart contracts, faster.
Need help with your project or have questions? Contact us via this form, on Twitter @QuickNode, or ping us on Discord!
About QuickNode
QuickNode is building infrastructure to support the future of Web3. Since 2017, we’ve worked with hundreds of developers and companies, helping scale dApps and providing high-performance access to 16+ blockchains. Subscribe to our newsletter for more content like this and stay in the loop with what’s happening in Web3! 😃