DeFi-SDK — A Beginner’s Guide
Integrating with different Ethereum protocols and performing common tasks is cumbersome. Today we will explore DeFi-SDK and how it simplifies working with several DeFi protocols in parallel.
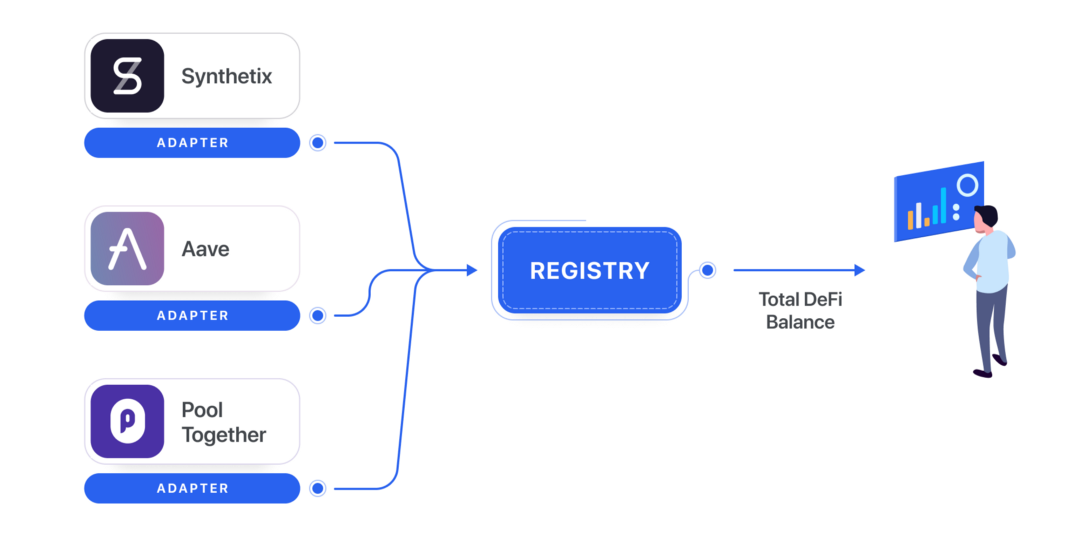
DeFi Programming, Now Easier Than Ever
Integrating with different Ethereum protocols and performing common tasks is cumbersome. For example, you need to get smart-contract addresses and ABI's for various DeFi protocols, and then manage all of them.
To solve this problem, the Zerion team launched DeFi SDK. Today we will talk about how it simplifies integrating different DeFi protocols.
What is DeFi-SDK?
DeFi-SDK is a set of smart contracts and APIs to perform common tasks across Ethereum #DeFi protocols such as Compound and Aave. As per official docs:
“DeFi SDK is an open-source system of smart contracts that makes it easier for developers to integrate DeFi protocols.”
What problem does DiFi-SDK solve?
DeFi-SDK helps you to easily integrate several DeFi protocols, and standardizes the way you interact with them.
Supported Protocols
DeFi-SDK currently supports:
- PieDAO
- Multi-Collateral Dai
- Bancor
- DeFi Money Market
- TokenSets
- 0x Staking
- Uniswap V1
- Synthetix
- PoolTogether
- Dai Savings Rate
- Chai
- iearn.finance (v2, v3)
- Idle
- dYdX
- Curve
- Compound
- Balancer
- Aave
Install DeFi-SDK
First, we will create a new directory:
mkdir defi-sdk
Currently, DeFi-SDK is available in Python and Go language only. Today, we will use the Python version on an Ubuntu system. defisdk
requires Python 3.8, so let’s install that.
Install Python 3.8 developer package:
sudo apt-get install python3.8-dev
Now install defisdk
:
python3.8 -m pip install defisdk --no-cache-dir
If you encounter an error, jump in the Zerion discord group.
Working with DeFi-SDK
Now, let’s understand how defisdk
works with some examples.
We will create a file index.py
in our defi-sdk
directory:
touch index.py
Next open this file, we will be using it for the defisdk
examples.
Initial Setup
We need to initialize our defisdk
. For that, we require an ETH MainNet web3 endpoint URL.
You can sign up for QuickNode and get the URL.
import asyncio
from defisdk import DeFiSDK
ETHEREUM_NODE_URL = 'YOUR_QUICKNODE_URL'
defi_sdk = DeFiSDK(ETHEREUM_NODE_URL)
You can see in line number 2,3,4 we are importing the SDK and creating an object by passing an Ethereum node URL.
In the first line, we are importing a library which we will use later.
Note: If you are building an application for use in production, a service like QuickNode can save you time, money, and help make your app faster.
Getting All the Protocols
We have done the initial set up.
Now we will get all the protocols supported by the defisdk
:
async def get_protocols():
print(await defi_sdk.get_protocol_names())
asyncio.run(get_protocols())
Here, defi_sdk.get_protocol_names()
will give us a protocol name, it's an asynchronous function because it calls smart-contracts under the hood.
Add the above lines in the same file (index.py
).
Now we will run our file to see the result:
python3.8 index.py
This will output all the supported protocol by defisdk
:

Get Balance from All Protocols
In this example, we will get the token balance of a user from all protocols in a single call.
USER_ADDRESS = '0xa218a8346454c982912cf6d14c714663c2d510d8'
async def get_account_balance():
balance = await defi_sdk.get_account_balance(USER_ADDRESS)
print(balance)
asyncio.run(get_account_balance())
Here, defi_sdk.get_account_balance(USER_ADDRESS)
is the function which will get us the user balance across protocols.
Add the above lines to our index.py
and let’s run it:
python3.8 index.py

You can check out the complete documentation of the DeFi SDK to learn more about it.
DeFi-SDK Architecture
Now, let’s understand the DeFi-SDK architecture.
defisdk
is a combination of two things:
1- Smart-contract adapters + a registry
2- A library written in python calling Defi SDK smart-contract functions
There are two types of Adapters:
- ProtocolAdapter: a special contract for every protocol. Its main purpose is to wrap all the protocol interactions. There are different types of protocol adapters: “Asset” adapter returns the amount of the account’s tokens held on the protocol, and the “Debt” adapter returns the amount of the account’s debt to the protocol. Some protocols do not use “simple” ERC20 tokens but instead have complex derivatives (for example the Compound protocol has
CTokens
). The ProtocolAdapter contract also provides information about the type of tokens used within it. - TokenAdapter: a contract for every derivative token type (eg. cTokens, aTokens, yTokens, etc...). Its main purpose is to provide ERC20-style token metadata as well as information about the underlying ERC20 tokens (like DAI for cDAI). Namely, it provides addresses, types and rates of underlying tokens.
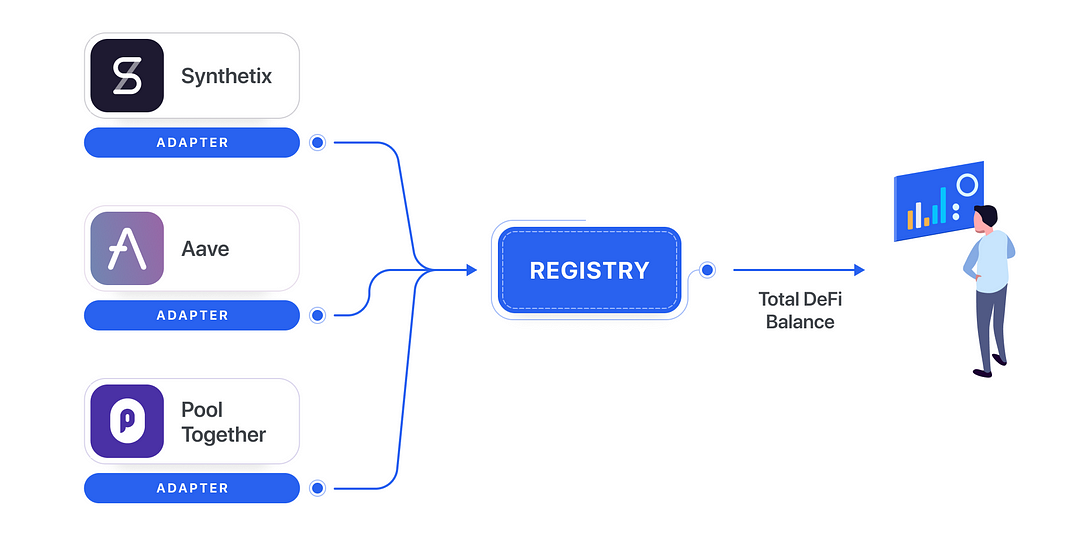
Wrapping Up
Working with multiple protocols can still be quite challenging in the Ethereum ecosystem, but thanks to some new libraries, companies are trying to solve this problem. In future articles, we will see similar libraries.
Need help with your project or have questions? Contact us via this form, on Twitter @QuickNode, or ping us on Discord!
About QuickNode
QuickNode is building infrastructure to support the future of Web3. Since 2017, we’ve worked with hundreds of developers and companies, helping scale dApps and providing high-performance access to 16+ blockchains. Subscribe to our newsletter for more content like this and stay in the loop with what’s happening in Web3! 😃